C program for solving quadratic equation 1. C program to calculate roots of a quadratic equation. I am using Dev C compiler.i have the C code to solve a quadratic equation.the.exe file runs very well and provide the required solutions.my problem is that i want the program to function in a gui environment still using C language and the. Feb 24, 2008 In case anyone reading this doesn't know, quadratic equation is: x = (-b +- sqrt((b^2)-4ac))/2a Now my question is, how would I put that into C in my program? (I haven't started it yet because I want to figure out how to write this equation into it first) Thanks! Like, Comments, Share and SUBSCRIBEvisit www.mysirg.com for all FREE videos.
The C++ program to solve quadratic equations in standard form is a simple program based on the quadratic formula. Given the coefficients as input, it will solve the equation and output the roots of the equation.
This is a simple program is intended for intermediate level C++ programmers.
The program is compiled using Dev-C++ 4.9.9.2 version installed on a Windows 7 64-bit PC. You may try other standard C compilers and the program will still work if you use the correct C libraries.
First, realize that the program won't ask you to 'insert a quadratic equation.' That would be useless. It will ask for the A, B and C factors, then compute the roots using the Quadratic Formula. X = -b ± √( b² - 4. a. c ) / ( 2. a ) Other than checking for imaginary roots (where b² - 4. a. c is negative), this is fall-through coding. Quadratic Formula Dev C++ Girls Games Cooking Download 3utools Stuck At Enter Recovery Mode B4 2 Vst Download Antares Auto Tune Pro Manual Does Auto Tune Ever Go On Sale Tinkertools Github Refx Serum Crack Auto Tune Pedal Review D850 Auto Fine Tune Little Snitch 3.7 2.
Problem Definition
In this program, we solve the quadratic equation using the formula
When
Where a, b and c are coefficient of the equation which is in the standard form.
How to compute?
The steps to compute the quadratic equation is given below.
Step1:
Quadratic Formula Dev C Everarts C
The program request the input coefficient values a, b and c. When the user input the values, it will compute two terms t1 and t3 and an intermediate term t2.
Step2:
The function term2 ()
is called in step 2 and returned value of function is assigned to t2
. The term2 ()
function receives the coefficient values – a, b, c and compute the value for t2.
The term ()
function returns and assign value of b2 – 4ac
to t2
and it is useful in understanding the root of the quadratic equation.
For example,
If (t2 < 0), then the roots are not real
If (t2 0) then, there is exactly one root value.
If (t2 > 0) then there are two root values.
The above condition is checked and printed with output values. Now we need to compute the roots and display the output values.
Step3:
A term t3
is assigned value after taking square root of t2.
Step4:
Finally, we have t1 and t3 to compute two roots of a quadratic equation.
Then root1
and root
are calculated and printed immediately.
Flowchart – Program for Quadratic Equations
To understand flow of logic of this program, see the flowchart below.

Program Code – Program for Quadratic Equation
Output
The output of the above program is given below.
I have given here a C program to solve any Quadratic Equation. Quadratic equation is a second order of polynomial equation in a single variable.
x = [ -b +/- sqrt(b^2 - 4ac) ] / 2a
We have to find the value of (b*b - 4*a*c).
- When it is greater than Zero, we will get two Real Solutions.
- When it is equal to zero, we will get one Real Solution.
- When it is less than Zero, we will get two Imaginary Solutions.
When there is an imaginary solutions, we have to use the factor i to represent imaginary part as it is a complex number.
Source Code
#include<math.h>
#include<stdio.h>
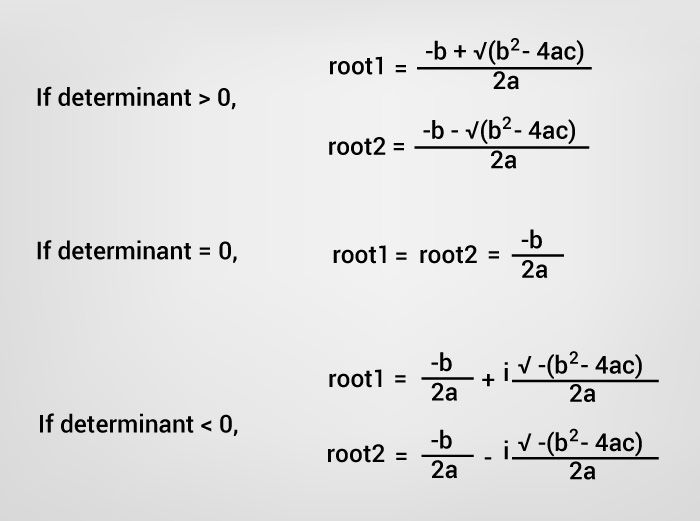
// quadratic equation is a second order of polynomial equation in a single variable
// x = [ -b +/- sqrt(b^2 - 4ac) ] / 2a
void SolveQuadratic(double a, double b, double c)
{
double sqrtpart = b*b - 4*a*c;
double x, x1, x2, img;
if(sqrtpart > 0)

{
x1 = (-b + sqrt(sqrtpart)) / (2 * a);
x2 = (-b - sqrt(sqrtpart)) / (2 * a);
printf('Two Real Solutions: %.4lf or %.4lfnn', x1, x2);
}
elseif(sqrtpart < 0)
Dev C++ Online
{
sqrtpart = -sqrtpart;
x = -b / (2 * a);
img = sqrt(sqrtpart) / (2 * a);
printf('Two Imaginary Solutions: %.4lf + %.4lf i or %.4lf + %.4lf inn', x, img, x, img);
}
else
{
x = (-b + sqrt(sqrtpart)) / (2 * a);
printf('One Real Solution: %.4lfnn', x);
}
}
int main()
{
// 6x^2 + 11x - 35 = 0
SolveQuadratic(6, 11, -35);

// 5x^2 + 6x + 1 = 0
SolveQuadratic(5, 6, 1);
Dev C++ For Windows 10
// 2x^2 + 4x + 2 = 0
SolveQuadratic(2, 4, 2);
// 5x^2 + 2x + 1 = 0
SolveQuadratic(5, 2, 1);
return 0;
}Output
Two Real Solutions: 1.6667 or -3.5000
Two Real Solutions: -0.2000 or -1.0000
One Real Solution: -1.0000
Two Imaginary Solutions: -0.2000 + 0.4000 i or -0.2000 + 0.4000 i
Press any key to continue . . .